AffinityMatcher
- class torchdr.AffinityMatcher(affinity_in: Affinity, affinity_out: Affinity, kwargs_affinity_out: dict = {'log': True}, n_components: int = 2, loss_fn: str = 'square_loss', kwargs_loss: dict = {'log': True}, optimizer: str = 'Adam', optimizer_kwargs: dict | None = None, lr: float | str = 1.0, scheduler: str = 'constant', scheduler_kwargs: dict | None = None, tol: float = 1e-07, max_iter: int = 1000, init: str | Tensor | ndarray = 'pca', init_scaling: float = 0.0001, tolog: bool = False, device: str = 'auto', keops: bool = False, verbose: bool = False, random_state: float = 0)[source]
Bases:
DRModule
Perform dimensionality reduction by matching two affinity matrices.
It amounts to solving a problem of the form:
\[\min_{\mathbf{Z}} \: \mathcal{L}( \mathbf{A_X}, \mathbf{A_Z})\]where \(\mathcal{L}\) is a loss function, \(\mathbf{A_X}\) is the input affinity matrix and \(\mathbf{A_Z}\) is the affinity matrix of the embedding.
The embedding optimization is performed using a first-order optimization method, with gradients calculated via PyTorch’s automatic differentiation.
- Parameters:
affinity_in (Affinity) – The affinity object for the input space.
affinity_out (Affinity) – The affinity object for the output embedding space.
kwargs_affinity_out (dict, optional) – Additional keyword arguments for the affinity_out method.
n_components (int, optional) – Number of dimensions for the embedding. Default is 2.
optimizer (str, optional) – Optimizer to use for the optimization. Default is “Adam”.
optimizer_kwargs (dict, optional) – Additional keyword arguments for the optimizer.
lr (float or 'auto', optional) – Learning rate for the optimizer. Default is 1e0.
scheduler (str, optional) – Learning rate scheduler. Default is “constant”.
scheduler_kwargs (dict, optional) – Additional keyword arguments for the scheduler.
tol (float, optional) – Tolerance for stopping criterion. Default is 1e-7.
max_iter (int, optional) – Maximum number of iterations. Default is 1000.
init (str | torch.Tensor | np.ndarray, optional) – Initialization method for the embedding. Default is “pca”.
init_scaling (float, optional) – Scaling factor for the initial embedding. Default is 1e-4.
tolog (bool, optional) – If True, logs the optimization process. Default is False.
device (str, optional) – Device to use for computations. Default is “auto”.
keops (bool, optional) – Whether to use KeOps for computations. Default is False.
verbose (bool, optional) – Verbosity of the optimization process. Default is False.
random_state (float, optional) – Random seed for reproducibility. Default is 0.
- fit(X: Tensor | ndarray, y=None)[source]
Fit the model to the provided data.
- Parameters:
X (torch.Tensor or np.ndarray of shape (n_samples, n_features)) – or (n_samples, n_samples) if precomputed is True Input data.
y (None) – Ignored.
- Returns:
self – The fitted AffinityMatcher instance.
- Return type:
- fit_transform(X: Tensor | ndarray, y=None)[source]
Fit the model to the provided data and returns the transformed data.
- Parameters:
X (torch.Tensor or np.ndarray of shape (n_samples, n_features)) – or (n_samples, n_samples) if precomputed is True Input data.
y (None) – Ignored.
- Returns:
embedding_ – The embedding of the input data.
- Return type:
Examples using AffinityMatcher
:
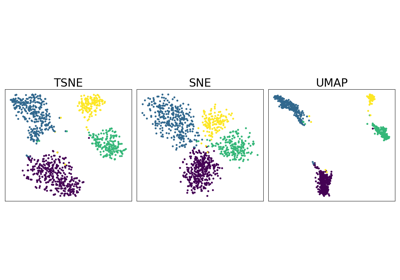
Neighbor Embedding on genomics & equivalent affinity matcher formulation